An example of extending the lifetime of a temporary:
#include <iostream>
int main()
{
int a = 1;
int&& b = std::move(a);
int&& c = b + 1;
b = b + 1;
c = c + 1;
std::cout << a << b << c;
}
The output is:
223
An example of dangling references:
#include <iostream>
#include <string>
const std::string& f() { return "abc"; }
std::string&& g() { return "xyz"; }
int main()
{
//Segmentation fault
const std::string s1 = f();
//Segmentation fault
std::string s2 = g();
s2 += "-uvw";
std::cout << s1 << ", " << s2 << std::endl;
return 0;
}
Explanation


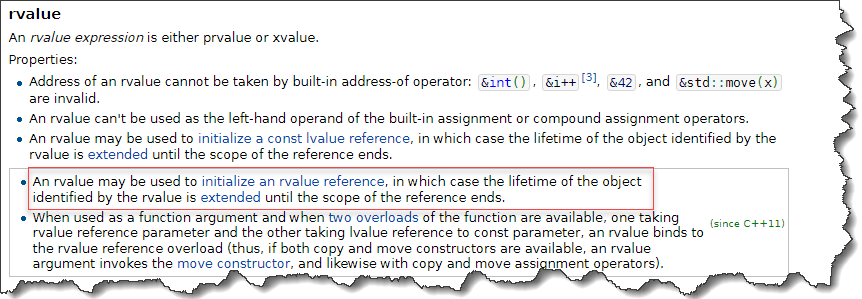
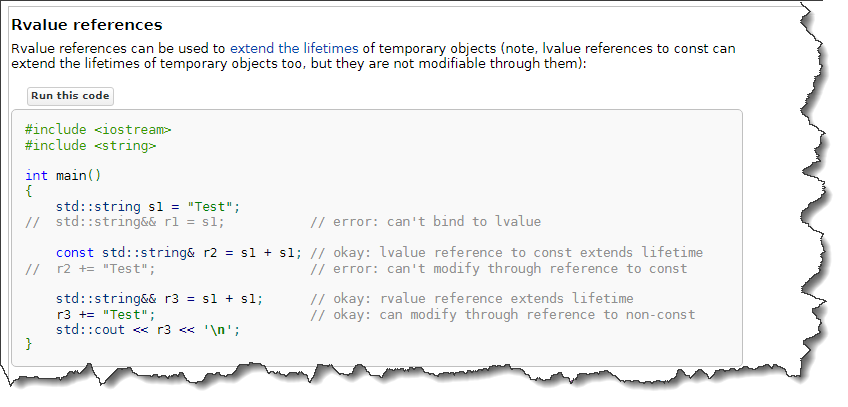
It is interesting that a function call can be a reference intialization:
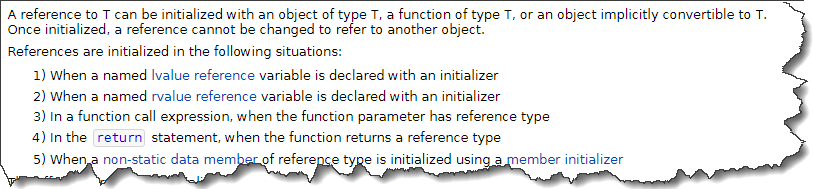