First I created a PHP file lines-app-store.php
on sharlines.com website with the following content:
<?php
$iPod = stripos($_SERVER['HTTP_USER_AGENT'],"iPod");
$iPhone = stripos($_SERVER['HTTP_USER_AGENT'],"iPhone");
$iPad = stripos($_SERVER['HTTP_USER_AGENT'],"iPad");
$macOs = stripos($_SERVER['HTTP_USER_AGENT'],"Mac");
$Android= stripos($_SERVER['HTTP_USER_AGENT'],"Android");
//check if user is using ipod, iphone or ipad...
if( $iPod || $iPhone || $iPad || $macOs)
{
//we send these people to Apple Store
header('Location: https://apps.apple.com/app/id6504194961');
}
else if($Android)
{
//we send these people to Android Store
header('Location: https://play.google.com/store/apps/details?id=net.geographx.LinesGame');
}
else
{
//we send these people to Windows Store
header('Location: https://www.microsoft.com/ru-ru/store/p/lines-3d/9nblggh5g9c6');
}
?>
Then I installed pip
in my Ubuntu 22.04 WSL and executed the following Python script:
# Importing library
import qrcode
# Data to be encoded
data = 'https://sharlines.com/lines-app-store.php'
# Encoding data using make() function
img = qrcode.make(data)
# Saving as an image file
img.save('lines-qr.png')
sudo apt install python3-pip
pip3 install qrcode
python3 qr.py
and got the following QR code:
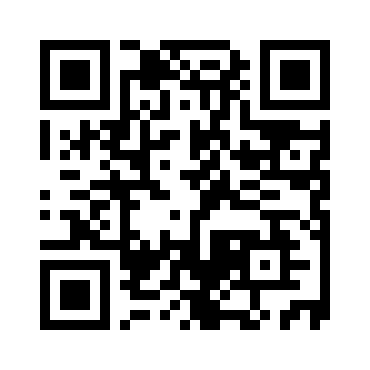
Generating SVG:
# Importing library
import qrcode
import qrcode.image.svg
# Data to be encoded
data = 'https://sharlines.com/lines-app-store.php'
# Encoding data using make() function
img = qrcode.make(data, image_factory=qrcode.image.svg.SvgImage)
# Saving as an image file
img.save('lines-qr.svg')
PNG with minimal border:
# Importing library
import qrcode
# Data to be encoded
data = 'https://sharlines.com/lines-app-store.php'
# Encoding data using make() function
img = qrcode.make(data, border=1)
# Saving as an image file
img.save('lines-qr.png')
How to make QR code for BOTH Android Market and App Store
https://stackoverflow.com/questions/6531667/how-to-make-qr-code-for-both-android-market-and-app-store
with PHP code.
Generate QR Code using qrcode in Python
https://www.geeksforgeeks.org/generate-qr-code-using-qrcode-in-python/
pip install qrcode
Access WSL2/Ubuntu Drive from File Explorer
https://learn.microsoft.com/en-us/answers/questions/116126/access-wsl2-ubuntu-drive-from-file-explorer
Game Data directory on MacOS:
/Users/admin/Library/Containers/com.sharlines.balls/Data/Library/Application Support/Sharlines/Lines
Generating SVG:
https://stackoverflow.com/questions/56328890/generate-the-svg-with-constant-size-by-python-qrcode
Resizing using the qrcode and Pillow libraries:
https://medium.com/@rahulmallah785671/create-qr-code-by-using-python-2370d7bd9b8d