I followed this instruction and configured C/C++ extension as follows:
{
"configurations": [
{
"name": "Win32",
"includePath": [
"${workspaceFolder}/**"
],
"defines": [
"_DEBUG",
"UNICODE",
"_UNICODE"
],
"windowsSdkVersion": "10.0.19041.0",
"compilerPath": "D:\\dev\\tools\\x86_64-12.2.0-release-win32-seh-rt_v10-rev0\\mingw64\\bin\\g++.exe",
"cStandard": "c17",
"cppStandard": "c++17",
"intelliSenseMode": "windows-gcc-x64"
}
],
"version": 4
}
then I created and successfully compiled helloworld.cpp
:
#include <iostream>
#include <vector>
#include <string>
using namespace std;
int main()
{
vector<string> msg {"Hello", "C++", "World", "from", "VS Code", "and the C++ extension!"};
for (const string& word : msg)
{
cout << word << " ";
}
cout << endl;
}
I also was able to run the program I compiled by pressing triangle button at top-right corner, and IntelliSense also worked:
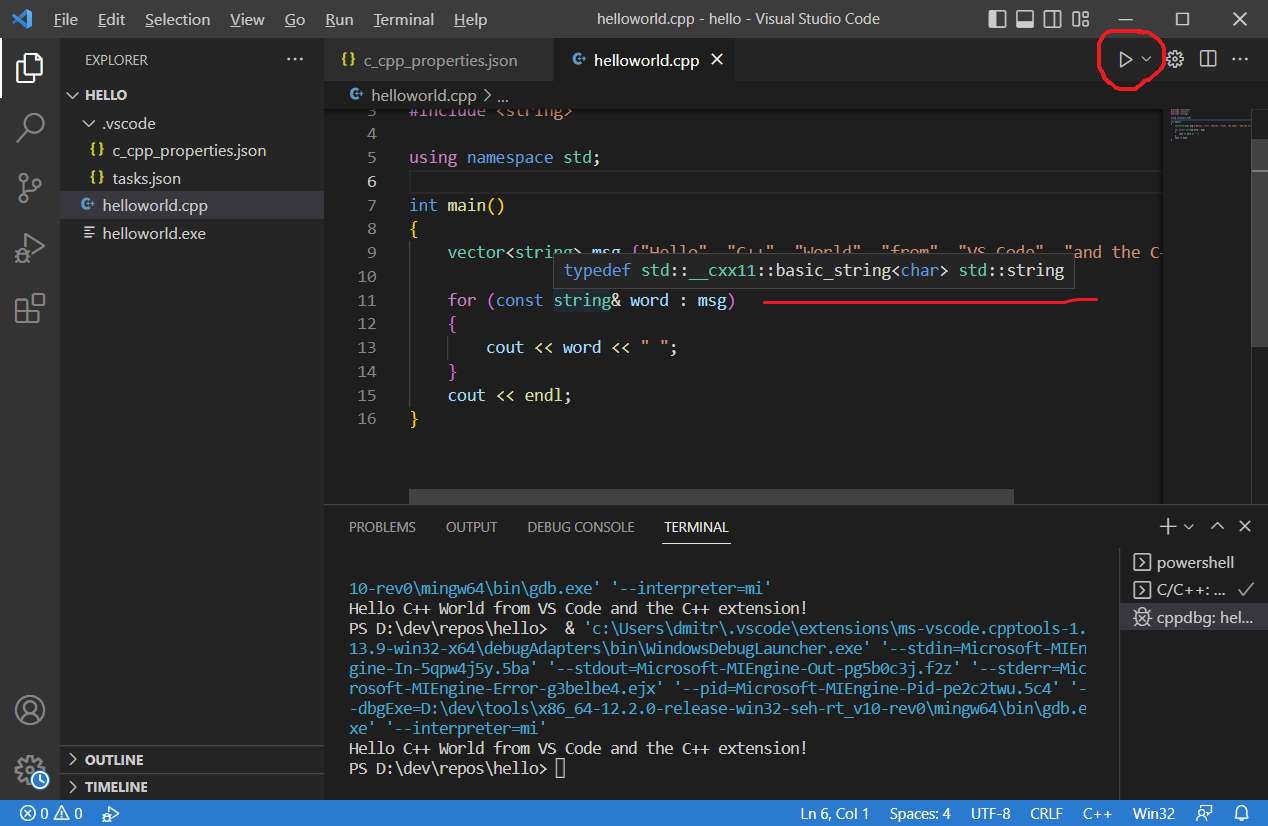
tasks.json
was created automatically:
{
"tasks": [
{
"type": "cppbuild",
"label": "C/C++: g++.exe build active file",
"command": "D:\\dev\\tools\\x86_64-12.2.0-release-win32-seh-rt_v10-rev0\\mingw64\\bin\\g++.exe",
"args": [
"-fdiagnostics-color=always",
"-g",
"${file}",
"-o",
"${fileDirname}\\${fileBasenameNoExtension}.exe"
],
"options": {
"cwd": "D:\\dev\\tools\\x86_64-12.2.0-release-win32-seh-rt_v10-rev0\\mingw64\\bin"
},
"problemMatcher": [
"$gcc"
],
"group": {
"kind": "build",
"isDefault": true
},
"detail": "Task generated by Debugger."
}
],
"version": "2.0.0"
}
My project tree looked like this at this point:
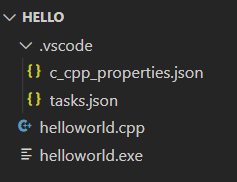
Then I set a breakpoint and it was triggered successfully when I started debug session:
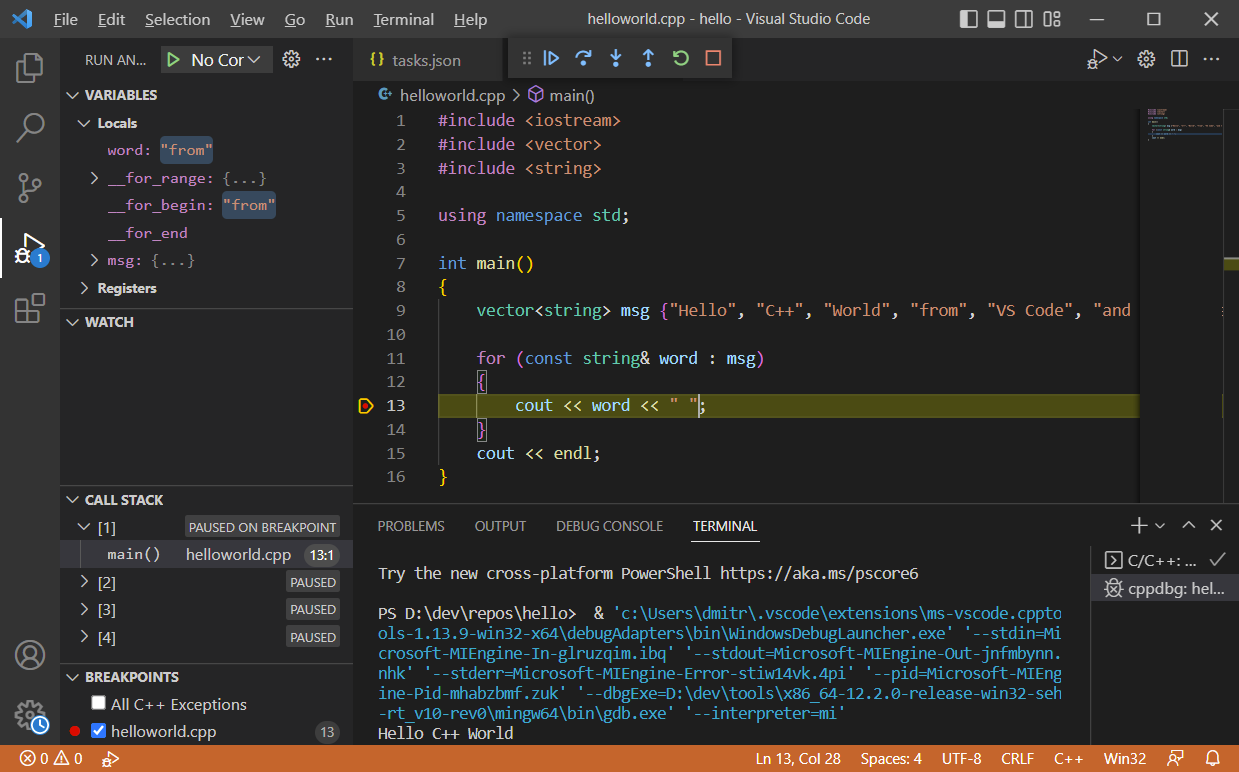
Alternatively we use commands:
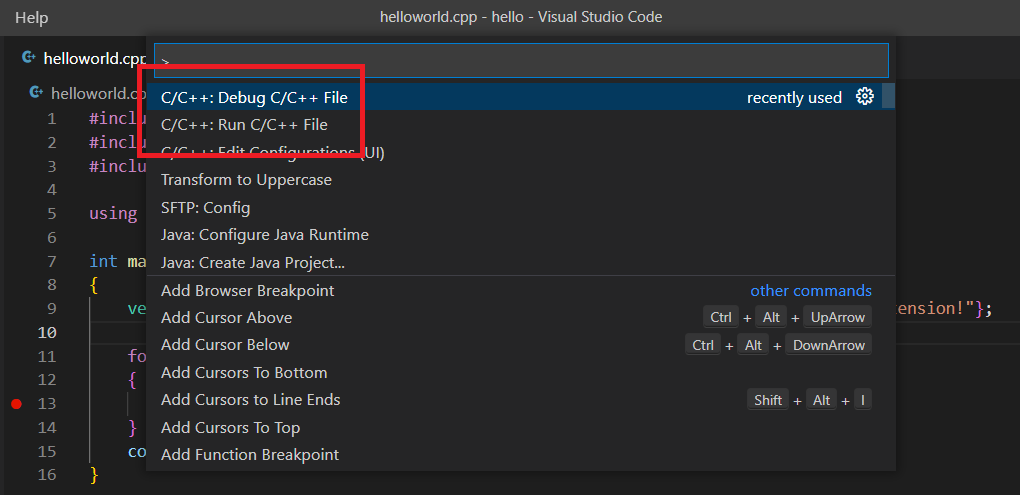
My plugin version:
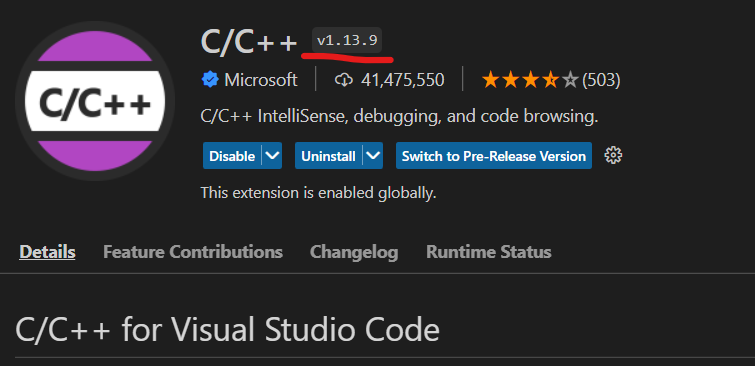
Using GCC with MinGW:
https://code.visualstudio.com/docs/cpp/config-mingw
Debug C++ in Visual Studio Code:
Windows debugging with GDB (“miDebuggerPath”: “c:\\mingw\\bin\\gdb.exe”)
https://code.visualstudio.com/docs/cpp/cpp-debug
Get started with CMake Tools on Linux:
https://code.visualstudio.com/docs/cpp/CMake-linux
Visual Studio Code CMake Tools ignores custom kits?
https://stackoverflow.com/questions/73915729/visual-studio-code-cmake-tools-ignores-custom-kits
How to configure VS Code’s CMake Tools Extension for GCC and MSYS Makefiles on Windows?
https://stackoverflow.com/questions/71000530/how-to-configure-vs-codes-cmake-tools-extension-for-gcc-and-msys-makefiles-on-w
https://stackoverflow.com/questions/3081815/c-errors-while-compiling
If you build the above code snippet with gcc you get the errors you mention. If you use g++ it build ok, this makes sense since g++ will make sure all the proper stuff it put together when build C++.
Tried:
set PATH=C:\WINDOWS\system32;C:\WINDOWS
set PATH=%PATH%;”D:\dev\tools\mingw64\bin”
and then run VS Code, but the kits still not found.
Using C++ and WSL in VS Code
https://code.visualstudio.com/docs/cpp/config-wsl
CMake Kit examples:
https://vector-of-bool.github.io/docs/vscode-cmake-tools/kits.html
https://github.com/microsoft/vscode-cmake-tools/blob/main/docs/kits.md