In CMake Tools extension settings I specified my CMake path:
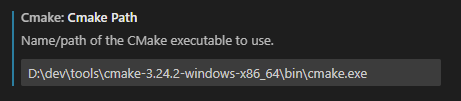
and built my project with MSVC 2022 first and then switched to MinGW.
Building a project with MSVC 2022
On my machine with installed MSVC 2022 it automatically generated configuration file %LocalAppData%/CMakeTools/cmake-tools-kits.json
:
[
{
"name": "Visual Studio Professional 2022 Release - amd64",
"visualStudio": "04bbaecf",
"visualStudioArchitecture": "x64",
"preferredGenerator": {
"name": "Visual Studio 17 2022",
"platform": "x64",
"toolset": "host=x64"
}
},
{
"name": "Visual Studio Professional 2022 Release - amd64_x86",
"visualStudio": "04bbaecf",
"visualStudioArchitecture": "x64",
"preferredGenerator": {
"name": "Visual Studio 17 2022",
"platform": "win32",
"toolset": "host=x64"
}
},
{
"name": "Visual Studio Professional 2022 Release - x86",
"visualStudio": "04bbaecf",
"visualStudioArchitecture": "x86",
"preferredGenerator": {
"name": "Visual Studio 17 2022",
"platform": "win32",
"toolset": "host=x86"
}
},
{
"name": "Visual Studio Professional 2022 Release - x86_amd64",
"visualStudio": "04bbaecf",
"visualStudioArchitecture": "x86",
"preferredGenerator": {
"name": "Visual Studio 17 2022",
"platform": "x64",
"toolset": "host=x86"
}
}
]
Then I opened a folder containing a project with CMakeLists.txt
selected Visual Studio Professional 2022 Release - amd64
kit and was able to build it with Debug
and RelWithDebInfo
variants:

Then I opened main.cpp
from the project, set a breakpoint, typed Ctrl+Shift+P
, selected CMake: Debug
and was able to debug the project:
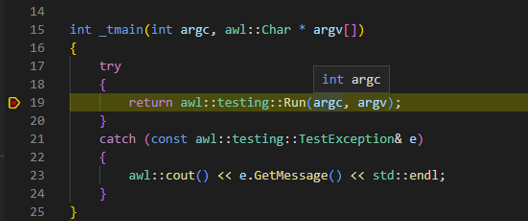
Switching to MinGW GCC kit
I manually added GCC compiler from MinGW into %LocalAppData%/CMakeTools/cmake-tools-kits.json
:
{
"name": "MinGW GCC",
"compilers": {
"C": "D:\\dev\\tools\\x86_64-12.2.0-release-win32-seh-rt_v10-rev0\\mingw64\\bin\\gcc.exe",
"CXX": "D:\\dev\\tools\\x86_64-12.2.0-release-win32-seh-rt_v10-rev0\\mingw64\\bin\\g++.exe"
}
}
and added .vscode/settings.json
file with the following content to project root folder:
{
"cmake.generator": "Ninja",
"cmake.configureSettings": {
"CMAKE_MAKE_PROGRAM": "D:\\dev\\tools\\ninja-win\\ninja.exe"
}
}
And my project started to build by I get strange compiler errors like:
[main] Building folder: Awl
[build] Starting build
[proc] Executing command: "C:\Program Files\Microsoft Visual Studio\2022\Professional\Common7\IDE\CommonExtensions\Microsoft\CMake\CMake\bin\cmake.exe" --build d:/dev/repos/Awl/build --config RelWithDebInfo --target all --
[build] [10/47 2% :: 1.259] Building CXX object CMakeFiles/AwlTest.dir/Tests/CancellationTest.cpp.obj
[build] FAILED: CMakeFiles/AwlTest.dir/Tests/CancellationTest.cpp.obj
[build] D:\dev\tools\x86_64-12.2.0-release-win32-seh-rt_v10-rev0\mingw64\bin\g++.exe -ID:/dev/repos/Awl -O2 -g -DNDEBUG -Wall -Wextra -pedantic -std=gnu++20 -MD -MT CMakeFiles/AwlTest.dir/Tests/CancellationTest.cpp.obj -MF CMakeFiles\AwlTest.dir\Tests\CancellationTest.cpp.obj.d -o CMakeFiles/AwlTest.dir/Tests/CancellationTest.cpp.obj -c D:/dev/repos/Awl/Tests/CancellationTest.cpp
[build] In file included from D:/dev/repos/Awl/Awl/Sleep.h:12,
[build] from D:/dev/repos/Awl/Tests/CancellationTest.cpp:9:
[build] D:/dev/repos/Awl/Awl/CppStd/Thread.h:21:45: error: 'condition_variable_any' in namespace 'std' does not name a type
[build] 21 | using condition_variable_any = std::condition_variable_any;
[build] | ^~~~~~~~~~~~~~~~~~~~~~
[build] D:/dev/repos/Awl/Awl/CppStd/Thread.h:4:1: note: 'std::condition_variable_any' is defined in header '<condition_variable>'; did you forget to '#include <condition_variable>'?
[build] 3 | #include <thread>
[build] +++ |+#include <condition_variable>
[build] 4 | #include <condition_variable>
[build] D:/dev/repos/Awl/Awl/Sleep.h: In function 'void awl::sleep_for(const std::chrono::duration<_Rep1, _Period1>&, const std::stop_token&)':
[build] D:/dev/repos/Awl/Awl/Sleep.h:19:14: error: 'condition_variable' is not a member of 'std'
[build] 19 | std::condition_variable cv;
[build] | ^~~~~~~~~~~~~~~~~~
[build] D:/dev/repos/Awl/Awl/Sleep.h:13:1: note: 'std::condition_variable' is defined in header '<condition_variable>'; did you forget to '#include <condition_variable>'?
[build] 12 | #include "Awl/CppStd/Thread.h"
[build] +++ |+#include <condition_variable>
[build] 13 |
[build] D:/dev/repos/Awl/Awl/Sleep.h:21:14: error: 'mutex' is not a member of 'std'
[build] 21 | std::mutex mutex;
[build] | ^~~~~
[build] D:/dev/repos/Awl/Awl/Sleep.h:13:1: note: 'std::mutex' is defined in header '<mutex>'; did you forget to '#include <mutex>'?
[build] 12 | #include "Awl/CppStd/Thread.h"
[build] +++ |+#include <mutex>
[build] 13 |
[build] D:/dev/repos/Awl/Awl/Sleep.h:23:31: error: 'mutex' is not a member of 'std'
[build] 23 | std::unique_lock<std::mutex> lock{ mutex };
[build] | ^~~~~
[build] D:/dev/repos/Awl/Awl/Sleep.h:23:31: note: 'std::mutex' is defined in header '<mutex>'; did you forget to '#include <mutex>'?
[build] D:/dev/repos/Awl/Awl/Sleep.h:23:36: error: template argument 1 is invalid
while all the headers were included.
And even IntelliSense know somehow that std::
was not defined:
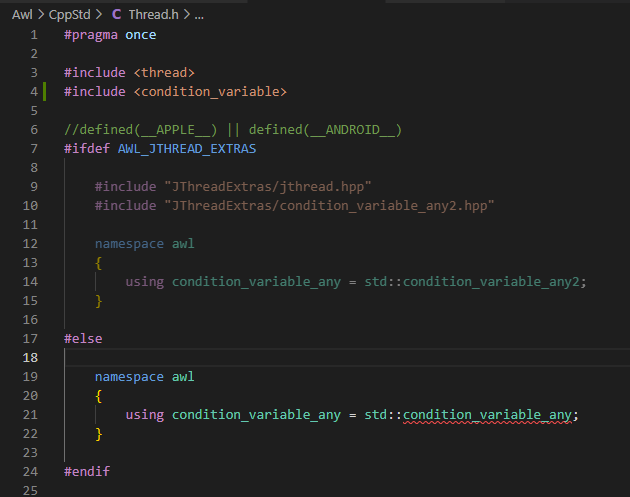
CMake command was the following:
D:\dev\tools\cmake-3.24.2-windows-x86_64\bin\cmake.exe --no-warn-unused-cli -DCMAKE_MAKE_PROGRAM:STRING=D:\dev\tools\ninja-win\ninja.exe ^
-DCMAKE_EXPORT_COMPILE_COMMANDS:BOOL=TRUE -DCMAKE_BUILD_TYPE:STRING=RelWithDebInfo -DCMAKE_C_COMPILER:FILEPATH=D:\dev\tools\x86_64-12.2.0-release-win32-seh-rt_v10-rev0\mingw64\bin\gcc.exe ^
-DCMAKE_CXX_COMPILER:FILEPATH=D:\dev\tools\x86_64-12.2.0-release-win32-seh-rt_v10-rev0\mingw64\bin\g++.exe -SD:/dev/repos/Awl -Bd:/dev/repos/Awl/build -G Ninja
CMake output:
[variant] Loaded new set of variants
[kit] Successfully loaded 5 kits from C:\Users\dmitr\AppData\Local\CMakeTools\cmake-tools-kits.json
[proc] Executing command: "D:\dev\tools\cmake-3.24.2-windows-x86_64\bin\cmake.exe " --version
[main] Configuring project: Awl
[proc] Executing command: "D:\dev\tools\cmake-3.24.2-windows-x86_64\bin\cmake.exe " --no-warn-unused-cli -DCMAKE_MAKE_PROGRAM:STRING=D:\dev\tools\ninja-win\ninja.exe -DCMAKE_EXPORT_COMPILE_COMMANDS:BOOL=TRUE -DCMAKE_BUILD_TYPE:STRING=RelWithDebInfo -DCMAKE_C_COMPILER:FILEPATH=D:\dev\tools\x86_64-12.2.0-release-win32-seh-rt_v10-rev0\mingw64\bin\gcc.exe -DCMAKE_CXX_COMPILER:FILEPATH=D:\dev\tools\x86_64-12.2.0-release-win32-seh-rt_v10-rev0\mingw64\bin\g++.exe -SD:/dev/repos/Awl -Bd:/dev/repos/Awl/build -G Ninja
[cmake] Not searching for unused variables given on the command line.
[cmake] -- The C compiler identification is GNU 12.2.0
[cmake] -- The CXX compiler identification is GNU 12.2.0
[cmake] -- Detecting C compiler ABI info
[cmake] -- Detecting C compiler ABI info - done
[cmake] -- Check for working C compiler: D:/dev/tools/x86_64-12.2.0-release-win32-seh-rt_v10-rev0/mingw64/bin/gcc.exe - skipped
[cmake] -- Detecting C compile features
[cmake] -- Detecting C compile features - done
[cmake] -- Detecting CXX compiler ABI info
[cmake] -- Detecting CXX compiler ABI info - done
[cmake] -- Check for working CXX compiler: D:/dev/tools/x86_64-12.2.0-release-win32-seh-rt_v10-rev0/mingw64/bin/g++.exe - skipped
[cmake] -- Detecting CXX compile features
[cmake] -- Detecting CXX compile features - done
[cmake] Awl C++ standard: 20
[cmake] -- Could NOT find Boost (missing: Boost_INCLUDE_DIR)
[cmake] BOOST not found, AWL will compile without BOOST.
[cmake] -- Performing Test CMAKE_HAVE_LIBC_PTHREAD
[cmake] -- Performing Test CMAKE_HAVE_LIBC_PTHREAD - Failed
[cmake] -- Looking for pthread_create in pthreads
[cmake] -- Looking for pthread_create in pthreads - not found
[cmake] -- Looking for pthread_create in pthread
[cmake] -- Looking for pthread_create in pthread - found
[cmake] -- Found Threads: TRUE
[cmake] -- Configuring done
[cmake] -- Generating done
[cmake] -- Build files have been written to: D:/dev/repos/Awl/build
To make CMake run from Windows Command Prompt I added CMAKE_RC_COMPILER:FILEPATH
to the command line:
D:\dev\tools\cmake-3.24.2-windows-x86_64\bin\cmake.exe --no-warn-unused-cli -DCMAKE_MAKE_PROGRAM:STRING=D:\dev\tools\ninja-win\ninja.exe ^
-DCMAKE_EXPORT_COMPILE_COMMANDS:BOOL=TRUE -DCMAKE_BUILD_TYPE:STRING=RelWithDebInfo -DCMAKE_C_COMPILER:FILEPATH=D:\dev\tools\x86_64-12.2.0-release-win32-seh-rt_v10-rev0\mingw64\bin\gcc.exe ^
-DCMAKE_CXX_COMPILER:FILEPATH=D:\dev\tools\x86_64-12.2.0-release-win32-seh-rt_v10-rev0\mingw64\bin\g++.exe -SD:/dev/repos/Awl -Bd:/dev/repos/Awl/build1 -G Ninja ^
-DCMAKE_RC_COMPILER:FILEPATH=D:\dev\tools\x86_64-12.2.0-release-win32-seh-rt_v10-rev0\mingw64\bin\windres.exe
CMake output from the Command Prompt was the following (build1
folder):
-- The C compiler identification is GNU 12.2.0
-- The CXX compiler identification is GNU 12.2.0
-- Detecting C compiler ABI info
-- Detecting C compiler ABI info - done
-- Check for working C compiler: D:/dev/tools/x86_64-12.2.0-release-win32-seh-rt_v10-rev0/mingw64/bin/gcc.exe - skipped
-- Detecting C compile features
-- Detecting C compile features - done
-- Detecting CXX compiler ABI info
-- Detecting CXX compiler ABI info - done
-- Check for working CXX compiler: D:/dev/tools/x86_64-12.2.0-release-win32-seh-rt_v10-rev0/mingw64/bin/g++.exe - skipped
-- Detecting CXX compile features
-- Detecting CXX compile features - done
Awl C++ standard: 20
-- Could NOT find Boost (missing: Boost_INCLUDE_DIR)
BOOST not found, AWL will compile without BOOST.
-- Performing Test CMAKE_HAVE_LIBC_PTHREAD
-- Performing Test CMAKE_HAVE_LIBC_PTHREAD - Failed
-- Looking for pthread_create in pthreads
-- Looking for pthread_create in pthreads - not found
-- Looking for pthread_create in pthread
-- Looking for pthread_create in pthread - found
-- Found Threads: TRUE
-- Configuring done
-- Generating done
-- Build files have been written to: D:/dev/repos/Awl/build1
Tried to build the project from Windows Command Prompt:
D:\dev\tools\cmake-3.24.2-windows-x86_64\bin\cmake.exe --build d:/dev/repos/Awl/build1 --config RelWithDebInfo --target all --
and got the same compiler errors.
I created a minimal example and was able to build it and even to debug it:
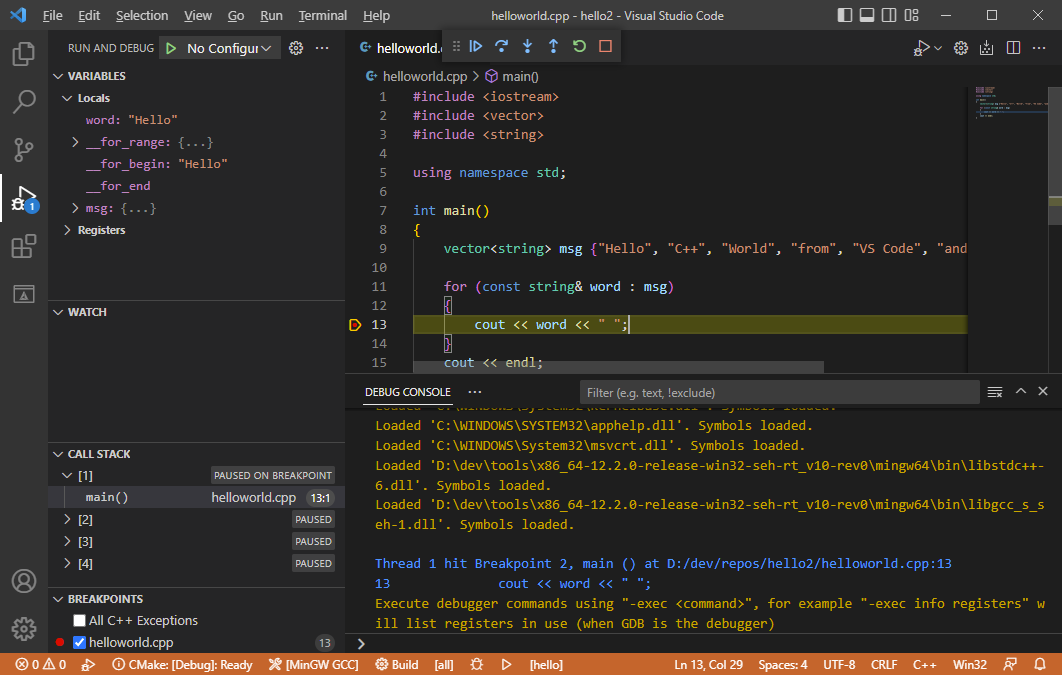
Note that I used Ctrl+Shift+P
and CMake: debug
command to start debugging.
An alternative way
Set PATH
and run VS Code:
set PATH=d:\dev\tools\mingw64\bin;d:\dev\tools\cmake\bin;C:\Users\<user name>\AppData\Local\Programs\Python\Python311;%PATH%
vscode.exe
Open project folder, locate CMakeLists.txt
, select the kit and target from the status bar:

MinGW kit automatically appears in the drop-down list.
settings.json
file with the following content will be created:
{
"cmake.sourceDirectory": "${workspaceFolder}/located/cmake-lists"
}
Debugger works better with white triangle button at the left side:
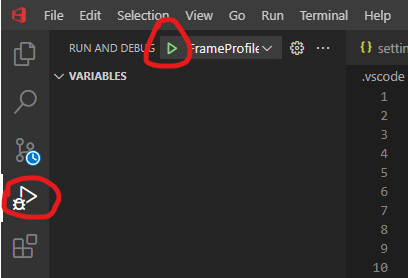
it creates launch.json
:
{
// Use IntelliSense to learn about possible attributes.
// Hover to view descriptions of existing attributes.
// For more information, visit: https://go.microsoft.com/fwlink/?linkid=830387
"version": "0.2.0",
"configurations": [
{
"name": "My Application",
"type": "cppdbg",
"request": "launch",
"program": "${workspaceFolder}/build/bin/MyApp.exe",
"args": [],
"stopAtEntry": false,
"cwd": "${fileDirname}",
"environment": [],
"externalConsole": false,
"MIMode": "gdb",
"targetArchitecture": "x64",
"setupCommands": [
{
"description": "Enable pretty-printing for gdb",
"text": "-enable-pretty-printing",
"ignoreFailures": true
}
]
}
]
}
About Ninja generator:
https://github.com/microsoft/vscode-cmake-tools/issues/134
About CMAKE_RC_COMPILER:FILEPATH:
https://stackoverflow.com/a/12853597/2394762
Default CMake path was C:\Program Files\Microsoft Visual Studio\2022\Professional\Common7\IDE\CommonExtensions\Microsoft\CMake\CMake\bin\cmake.exe
https://stackoverflow.com/a/17986785/2394762
I have found that the mutex class is defined under the following #if guard:
#if defined(_GLIBCXX_HAS_GTHREADS) && defined(_GLIBCXX_USE_C99_STDINT_TR1)
Regretfully, _GLIBCXX_HAS_GTHREADS is not defined on Windows. The runtime support is simply not there.
https://stackoverflow.com/a/30849490/2394762
This has now been included in MingW (Version 2013072300). To include it you have to select the pthreads package in the MinGW Installation Manager.
https://stackoverflow.com/a/27612609/2394762
Mutex, at least, is not supported in ‘Thread model: win32’ of the Mingw-builds toolchains. You must select any of the toolchains with ‘Thread model: posix’. After trying with several versions and revisions (both architectures i686 and x86_64) I only found support in x86_64-4.9.2-posix-seh-rt_v3-rev1 being the thread model, IMO, the determining factor.
We also add DCMAKE_MAKE_PROGRAM to CMake configuraion on Kits tab in QT Creator Preferences dialog:
-DQT_QMAKE_EXECUTABLE:FILEPATH=%{Qt:qmakeExecutable}
-DCMAKE_PREFIX_PATH:PATH=%{Qt:QT_INSTALL_PREFIX}
-DCMAKE_C_COMPILER:FILEPATH=%{Compiler:Executable:C}
-DCMAKE_CXX_COMPILER:FILEPATH=%{Compiler:Executable:Cxx}
-DCMAKE_MAKE_PROGRAM:FILEPATH=d:\dev\tools\ninja\ninja.exe
And select Ninja as CMake generator.