Starting from C++14:
#include <string>
#include <memory>
int main()
{
std::unique_ptr<std::string> p = std::make_unique<std::string>("abc");
auto func = [p = std::move(p)]()
{
};
func();
return 0;
}
or
auto func = [p{ std::move(p) }]()
{
};
or
auto func = [p = std::make_unique<std::string>("abc")]()
{
};
Each capture creates a new type-deduced local variable inside the lambda:
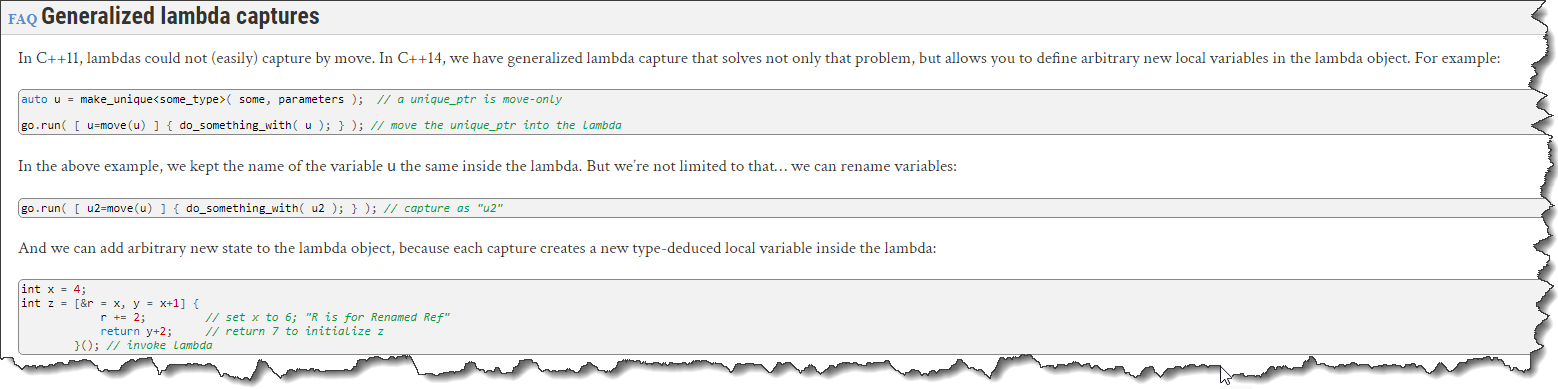