I run T-Rex miner as follows:
t-rex -a kawpow -o stratum+tcp://rvn.2miners.com:6060 -u <mywallet>.rigW -p x
and set the following parameters on my 1060 GTX 3GB card with MSI Afterburner:
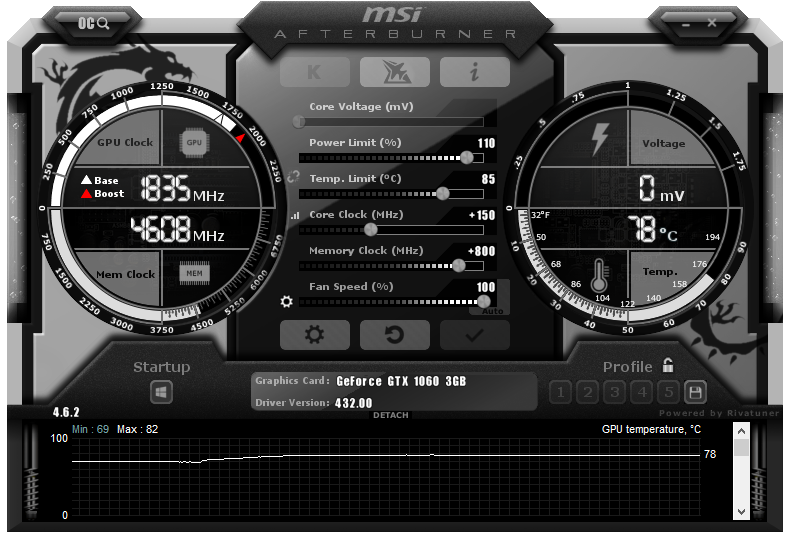
Check if unified auditing is enabled:
SELECT VALUE FROM V$OPTION WHERE PARAMETER = 'Unified Auditing';
Check what options are enabled:
SELECT up.AUDIT_OPTION, uep.SUCCESS, uep.FAILURE from AUDIT_UNIFIED_ENABLED_POLICIES uep, AUDIT_UNIFIED_POLICIES up
where uep.ENTITY_NAME = 'ALL USERS' and uep.ENABLED_OPTION='BY USER' and uep.POLICY_NAME = up.POLICY_NAME and up.AUDIT_OPTION_TYPE = 'STANDARD ACTION'
strawberry-perl-5.26.2.1-64bit.msi
first (before mingw-w64-install.exe
) to E:\PFiles
mingw-w64-install.exe
online installer. Select POSIX threads in the installer UI combobox, otherwise you will get:qt-everywhere-src-5.15.0.zip
to E:\Qt\Qt5.15.0
and run the following script in QT root directory containing configure.bat
:If I enable Gesture mode on an Android 10 emulator by tapping System->Gestures->System Navigation->Gesture Navigation and then deactivate my app by swiping and reactivate it back, I get the following in the log:
adb logcat | grep "\ Lines"
05-04 21:52:36.389 11794 11939 D Lines : Squircle::aboutToQuit()
05-04 21:52:36.389 11794 11939 D Lines : InterstitialAdWrapper clear() called.
05-04 21:52:36.392 11794 11939 D Lines : InterstitialAdWrapper InterstitialAd destructor called.
05-04 21:52:36.393 11794 11939 D Lines : Squircle destructor called.
05-04 21:52:36.396 11794 11939 W Lines : exit app 0
...
05-04 21:52:37.032 11794 11939 D Lines : OslSoundPool destructor
05-04 21:52:37.117 2040 2134 W InputDispatcher: channel '8474831 net.geographx.LinesGame/net.geographx.MainActivity (server)' ~ Consumer closed input channel or an error occurred. events=0x9
05-04 21:52:37.117 2040 2134 E InputDispatcher: channel '8474831 net.geographx.LinesGame/net.geographx.MainActivity (server)' ~ Channel is unrecoverably broken and will be disposed!
05-04 21:52:37.123 2040 3120 I WindowManager: WIN DEATH: Window{8474831 u0 net.geographx.LinesGame/net.geographx.MainActivity}
05-04 21:52:37.123 2040 3120 W InputDispatcher: Attempted to unregister already unregistered input channel '8474831 net.geographx.LinesGame/net.geographx.MainActivity (server)'
05-04 21:52:37.136 2040 3120 W ActivityManager: Scheduling restart of crashed service net.geographx.LinesGame/org.chromium.content.app.SandboxedProcessService0 in 1000ms
05-04 21:52:37.151 2040 2057 I ActivityManager: Process net.geographx.LinesGame (pid 11794) has died: cch CRE
05-04 21:52:38.034 2040 2057 I ActivityTaskManager: START u0 {act=android.intent.action.MAIN cat=[android.intent.category.LAUNCHER] flg=0x10200000 cmp=net.geographx.LinesGame/net.geographx.MainActivity bnds=[37,882][238,1191]} from uid 10090
05-04 21:52:38.055 2040 2057 I chatty : uid=1000(system) Binder:2040_2 identical 8 lines
05-04 21:52:38.099 2040 2072 I ActivityManager: Start proc 12702:net.geographx.LinesGame/u0a134 for activity {net.geographx.LinesGame/net.geographx.MainActivity}
05-04 21:52:38.101 12702 12702 W raphx.LinesGam: Unexpected CPU variant for X86 using defaults: x86
05-04 21:52:38.124 12702 12702 E raphx.LinesGam: Not starting debugger since process cannot load the jdwp agent.
...
05-04 21:52:38.966 2040 2069 I ActivityTaskManager: Displayed net.geographx.LinesGame/net.geographx.MainActivity: +931ms
05-04 21:52:39.062 12702 12826 D libLinesGameQt_x86.so: Translation file has been loaded successfully: "LinesGame_en"
05-04 21:52:39.062 12702 12826 D Lines : App version: "2.5.11, #133"
05-04 21:52:39.072 12702 12826 D Lines : QT/SysInfo: Device Pixel Ratio: 3 Screen DPI: 147.131
05-04 21:52:39.106 12702 12826 D Lines : InterstitialAdWrapper valid
05-04 21:52:39.185 12702 12826 D Lines : qml: Registering adfree
05-04 21:52:39.189 7632 9145 W Finsky : [672] gcs.d(23): net.geographx.LinesGame: No account found.
05-04 21:52:39.411 12702 12826 D Lines : qml: adfree Component.onCompleted
05-04 21:52:39.413 12702 12826 D Lines : InterstitialAdWrapper: calling Java method ' initializeInterstitialAd ' from C++
05-04 21:52:39.413 12702 12826 D Lines : Starting the application event loop...
05-04 21:52:39.463 12702 12826 D Lines : onApplicationStateChanged(Qt::ApplicationActive)