I was able to define a Repeater that displays cryptocurrency market orders:
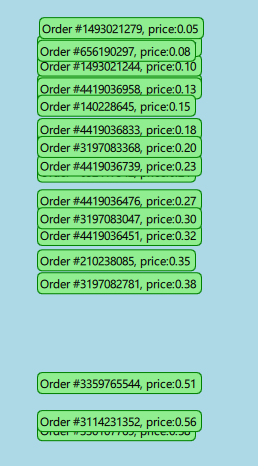
QML code is provided below:
import QtQuick
import QtQuick.Layouts
import QtQuick.Controls
import Qt.labs.qmlmodels
import TradeClient
Item
{
property real factor: 1.0
ColumnLayout
{
anchors.fill: parent
anchors.margins: 5
spacing: 5
OrderFilter
{
id: filter
onChanged: {
list.model.fill()
list.forceLayout()
}
}
Rectangle
{
Layout.fillWidth: true
Layout.fillHeight: true
clip: true
color: "lightblue"
Repeater
{
id: list
anchors.fill: parent
delegate: Item
{
x: 100
y: list.height * model.item.triggerPrice * factor
width: label.width + 6
height: label.height + 6
Rectangle
{
id: rect
anchors.fill: parent
color: "lightgreen"
border.color: "green"
radius: 5
}
Text
{
id: label
anchors.left: parent.left
anchors.top: parent.top
anchors.leftMargin: 3
anchors.topMargin: 3
text: "Order #%1, price:%2 ".arg(model.item.id.toFixed(0)).arg(model.item.triggerPrice.toFixed(2))
}
MouseArea
{
anchors.fill: parent
drag.target: parent
hoverEnabled: true
onEntered: rect.color = "olive"
onExited: rect.color = "lightgreen"
}
}
}
}
Rectangle
{
Layout.fillWidth: true
height: 1
color: "gray"
}
Flow
{
Layout.alignment: Qt.AlignRight
spacing: 5
Text {
text: "%1 orders".arg(list.model.rowCount)
color: "green"
}
}
}
Component.onCompleted:
{
var m = appModel.createOrders("percentage")
m.filter = filter
list.model = m
}
MouseArea
{
anchors.fill: parent
propagateComposedEvents: true
onWheel: (e) =>
{
//console.log(JSON.stringify(e))
factor *= (1.0 + e.angleDelta.y / (8 * 15 * 10))
}
onClicked: (mouse) =>
{
console.log("clicked blue")
mouse.accepted = false
}
onPressed: (mouse) =>
{
console.log("Pressed blue")
mouse.accepted = false;
}
onReleased: (mouse) =>
{
console.log("Released blue");
mouse.accepted = false;
}
}
}