From QT’s Purchasing:
//SKProductsRequestDelegate
-(void)productsRequest:(SKProductsRequest *)request didReceiveResponse:(SKProductsResponse *)response
{
NSArray<SKProduct *> *products = response.products;
SKProduct *product = [products count] == 1 ? [[products firstObject] retain] : nil;
if (product == nil) {
//Invalid product ID
NSString *invalidId = [response.invalidProductIdentifiers firstObject];
QMetaObject::invokeMethod(backend, "registerQueryFailure", Qt::AutoConnection, Q_ARG(QString, QString::fromNSString(invalidId)));
} else {
//Valid product query
//Create a IosInAppPurchaseProduct
IosInAppPurchaseProduct *validProduct = new IosInAppPurchaseProduct(product, backend->productTypeForProductId(QString::fromNSString([product productIdentifier])));
if (validProduct->thread() != backend->thread()) {
validProduct->moveToThread(backend->thread());
QMetaObject::invokeMethod(backend, "setParentToBackend", Qt::AutoConnection, Q_ARG(QObject*, validProduct));
}
QMetaObject::invokeMethod(backend, "registerProduct", Qt::AutoConnection, Q_ARG(IosInAppPurchaseProduct*, validProduct));
}
[request release];
}
-(void)dealloc
{
[[SKPaymentQueue defaultQueue] removeTransactionObserver:self];
[pendingTransactions release];
[super dealloc];
}
IosInAppPurchaseBackend::~IosInAppPurchaseBackend()
{
[m_iapManager release];
}
void IosInAppPurchaseBackend::initialize()
{
m_iapManager = [[QT_MANGLE_NAMESPACE(InAppPurchaseManager) alloc] initWithBackend:this];
emit InAppPurchaseBackend::ready();
}
From QtAdMob:
class QtAdMobBannerIos : public IQtAdMobBanner
{
...
#if defined(__OBJC__)
__unsafe_unretained QtAdMobBannerDelegate* m_AdMob;
#else
void* m_AdMob;
#endif
...
};
QtAdMobBannerIos::QtAdMobBannerIos(QObject* parent)
: IQtAdMobBanner(parent)
, m_BannerSize(IQtAdMobBanner::Banner)
, m_LoadingState(Idle)
{
QtAdMobBannerDelegate *delegate = [[QtAdMobBannerDelegate alloc] initWithHandler:this];
m_AdMob = (__bridge QtAdMobBannerDelegate*)(__bridge_retained void*)delegate;
}
From stackoverflow.com:
copy Makes a copy of an object, and returns it with retain count of 1. If you copy an object, you own the copy. This applies to any method that contains the word copy where “copy” refers to the object being returned.
retain Increases the retain count of an object by 1. Takes ownership of an object.
release Decreases the retain count of an object by 1. Relinquishes ownership of an object.
XCode Settings
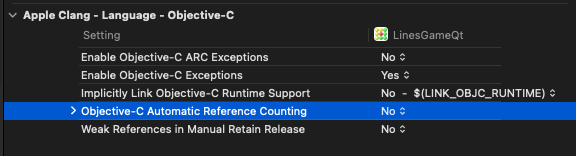
https://gist.github.com/garthhumphreys/2252898
garthhumphreys/NSLog-format-specifiers.txt
Objective-C – Private vs Protected vs Public
https://stackoverflow.com/questions/4869935/objective-c-private-vs-protected-vs-public
Destroy an object in objective-C
https://stackoverflow.com/questions/18301212/destroy-an-object-in-objective-c
@property (nonatomic, strong) Node* first;
@property (nonatomic, weak) Node* last;
Objective-C Memory Management with ARC
https://www.youtube.com/watch?v=EKf3t1ytZZs
My Question:
https://stackoverflow.com/questions/79023642/how-to-prevent-a-root-object-from-being-deleted-in-c-objective-c
yes, it is -fno-objc-arc compiler flag (stackoverflow.com/a/18883995/2394762)