Looks like CheckBox
-es respond to clicks and work correctly even if they are in a TableView
that is updated once a second:
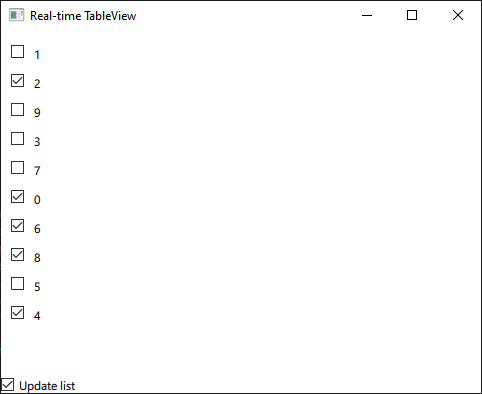
See QML source code below:
import QtQuick
import QtQuick.Window
import QtQuick.Controls
import QtQuick.Layouts
Window {
width: 640
height: 480
visible: true
title: qsTr("Real-time TableView")
ListModel
{
id: list
}
ColumnLayout
{
spacing: 5
anchors.fill: parent
TableView
{
id: table
model: list
columnSpacing: 5
rowSpacing: 3
clip: true
Layout.fillWidth: true
Layout.fillHeight: true
Layout.margins: 10
ScrollBar.horizontal: ScrollBar{}
ScrollBar.vertical: ScrollBar{}
ScrollIndicator.horizontal: ScrollIndicator { }
ScrollIndicator.vertical: ScrollIndicator { }
delegate: CheckBox
{
padding: 5
text: model.number
checked: model.checked
onClicked: model.checked = checked
}
}
CheckBox
{
text: "Update list"
checked: updateTimer.running
onClicked: updateTimer.running = checked
}
}
function randomIndex(max)
{
return Math.floor(Math.random() * max);
}
Timer {
id: updateTimer
interval: 1000;
running: true;
repeat: true
onTriggered:
{
var index1 = randomIndex(list.rowCount())
var index2 = randomIndex(list.rowCount())
console.log(index1, index2)
if (index1 !== index2)
{
list.move(index1, index2, 1)
}
}
}
Component.onCompleted:
{
for (var i = 0; i < 10; ++i)
{
var data = {'number': i, checked: i % 2 === 0};
list.append(data)
}
}
}
But if we change the timer code with the following:
Timer {
id: updateTimer
interval: 100;
running: true;
repeat: true
onTriggered:
{
var half = list.rowCount() / 2
var index1 = half + randomIndex(half)
var index2 = half + randomIndex(half)
console.log(index1, index2)
if (index1 !== index2)
{
list.move(index1, index2, 1)
}
}
}
we get an interesting effect: first five CheckBox
-es stops responding to the clicks despite the fact that they do not move.
QT version: 6.2.2