I was able to compile and debug my app on MacOS with QT Creator:
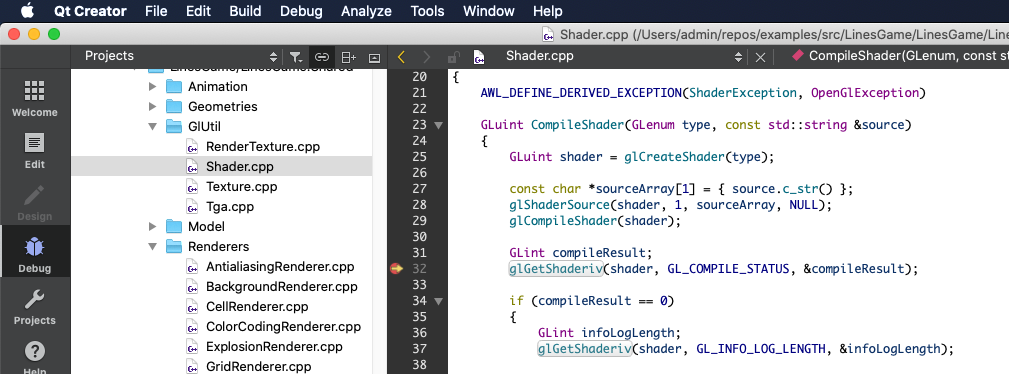
All C++ code compiled successfully, but something went wrong with OpenGLES shaders. It is probably because I included OpenGLES headers from a wrong place.
The following fragment shader did not compile:
//precision mediump float;
uniform vec4 u_color;
varying float v_lifetime;
uniform sampler2D s_texture;
void main()
{
vec4 texColor;
texColor = texture2D(s_texture, gl_PointCoord);
float a = texColor.r * texColor.g * texColor.b;
gl_FragColor = u_color;
gl_FragColor.a *= v_lifetime * a;
}
At first the error was with precision mediump float
, but after I commented it out, I got an error with gl_PointCoord
:
ERROR: 0:1: Use of undeclared identifier 'gl_PointCoord'
but fortunately it was the only shader that did not compile and finally I started my app without it:
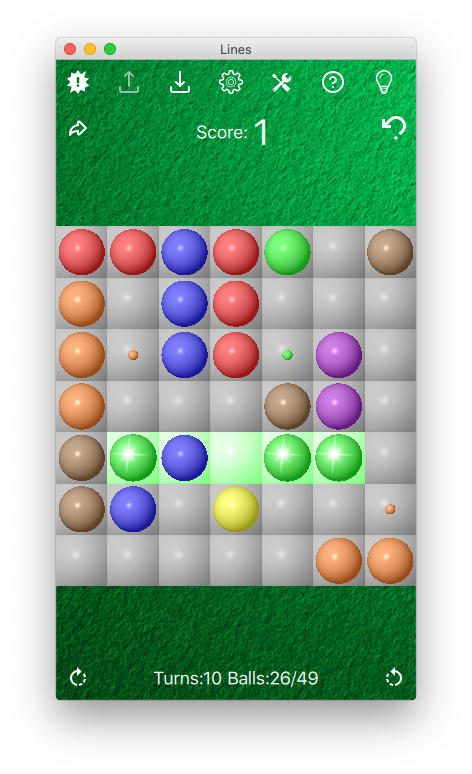
as you can see, antialiasing is not enabled yet.
To find OpenGLES headers I did a global search
sudo find / -iname "gl3.h"
and found /Applications/Xcode.app/Contents/Developer/Platforms/MacOSX.platform/Developer/SDKs/MacOSX.sdk/System/Library/Frameworks/OpenGL.framework/Versions/A/Headers/
directory containing the following headers:
-rw-r--r-- 1 root wheel 557 Feb 29 11:36 CGLContext.h
-rw-r--r-- 1 root wheel 467 Feb 29 11:36 CGLCurrent.h
-rw-r--r-- 1 root wheel 980 Feb 29 11:36 CGLDevice.h
-rw-r--r-- 1 root wheel 14632 Feb 29 11:36 CGLIOSurface.h
-rw-r--r-- 1 root wheel 134620 Feb 29 11:36 CGLMacro.h
-rw-r--r-- 1 root wheel 3135 Feb 29 11:36 CGLRenderers.h
-rw-r--r-- 1 root wheel 21090 Feb 29 11:36 CGLTypes.h
-rw-r--r-- 1 root wheel 6713 Feb 29 11:36 OpenGL.h
-rw-r--r-- 1 root wheel 775 Feb 29 11:36 OpenGLAvailability.h
-rw-r--r-- 1 root wheel 170748 Feb 29 11:36 gl.h
-rw-r--r-- 1 root wheel 182502 Feb 29 11:36 gl3.h
-rw-r--r-- 1 root wheel 14653 Feb 29 11:36 gl3ext.h
-rw-r--r-- 1 root wheel 217568 Feb 29 11:36 glext.h
-rw-r--r-- 1 root wheel 327 Feb 29 11:36 gliContext.h
-rw-r--r-- 1 root wheel 87660 Feb 29 11:36 gliDispatch.h
-rw-r--r-- 1 root wheel 1410 Feb 29 11:36 gltypes.h
-rw-r--r-- 1 root wheel 18745 Feb 29 11:36 glu.h
-rw-r--r-- 1 root wheel 3841 Feb 29 11:36 gluContext.h
-rw-r--r-- 1 root wheel 2838 Feb 29 11:36 gluMacro.h
Finally, I figured out that I include OpenGL on Desktop platform as follows:
#include <OpenGL/gl3.h>
#include <OpenGL/glu.h>
Compiling the app for iOS
To compile the app for iOS I included OpenGLES header as follows:
#include <OpenGLES/ES3/gl.h>
and all my code compiled, so I was able to start the app on the simulator:
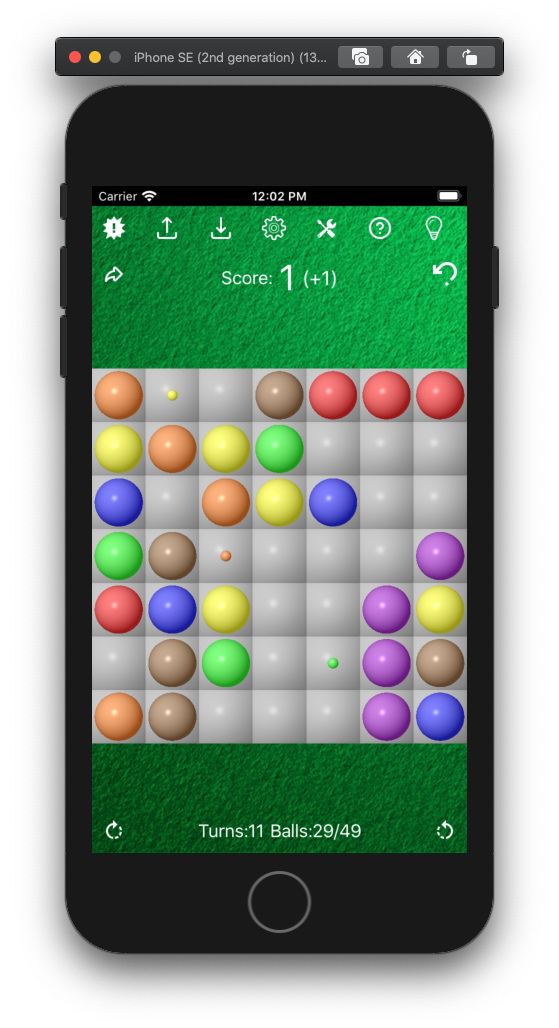
Notes about setting up the development environment
By the way I figured out what is the command key on my home-made Macintosh:

it is actually Windows key!
The only extremely annoying thing is that NumPad arrows does not work and this makes editing the text absolutely annoying, so I need to figure out how to fix this. The next significantly annoying thing is that I can’t use Ctrl+Ins, Shif+Ins, Shif+Del, etc… for working with the clipboard and select the text with Ctrl+Shift+ arrows.
QT installation path:
/Users/admin/Qt5.14.2
Running QT Creator from the terminal:
cd /Users/admin/Qt5.14.2/Qt\ Creator.app/Contents/MacOS
./Qt\ Creator &
Component versions
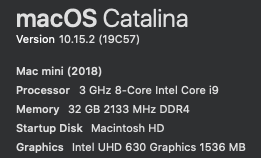
system_profiler SPDeveloperToolsDataType
Developer:
Developer Tools:
Version: 11.4.1 (11E503a)
Location: /Applications/Xcode.app
Applications:
Xcode: 11.4.1 (16137)
Instruments: 11.4.1 (64535.74)
SDKs:
iOS:
13,4: (17E8258)
iOS Simulator:
13,4: (17E8258)
macOS:
10.15.4: (19E258)
19,0:
tvOS:
13,4: (17L255)
tvOS Simulator:
13,4: (17L255)
watchOS:
6,2: (17T255)
watchOS Simulator:
6,2: (17T255)
OpenGLES/GLSL versions
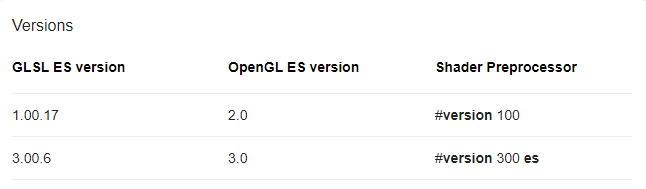
#if defined(__APPLE__)
#include <OpenGL/gl.h>
#include <OpenGL/glu.h>
#else
#include <GL/gl.h>
#include <GL/glu.h>
#endif
Links
- Keyboard Shortcuts to Capture a Screen Shot with Mac OS X.
- Take a screenshot on your Mac.
- How to make NumLock/numpad cursor keys work on an external USB keyboard?
- gl_PointCoord undeclared in fragment shader on macOS?
- gl_PointCoord support
- OpenGL Shading Language
- Qt: How to detect which version of OpenGL is being used?
- link OpenGL to Qt Creator under Mac osx
- c++ #ifdef Mac OS X question
- But it still outputs gl version 2.1 and glsl version 1.2
- What OpenGL version is available in a QT app on MacOS Desktop platform?
While compiling some other app I get “error: ‘any_cast’ is unavailable: introduced in macOS 10.14”
OpenGL version = 2.1 INTEL-14.3.9